- Back to Home »
- C plus »
- Difference between Binary Search and Linear Search
Posted by : UTariq
Thursday, September 26, 2013
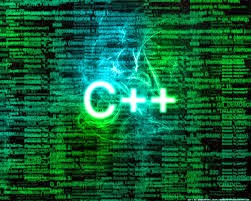
Linear Search:
Linear search is a very common method to search a number in array. In linear search , if there is 'n' numbers then we have to do 'n-1' comparison to search a particular number. It's a worst algorithm. The algorithm of linear search search a number at every index on array. For Example if there is a number on 9th Index, then first it check at index 1 then index 2 and vice versa. After 8th comparison we got our result.The Program of linear search is as :
#include<iostream>
#include<conio.h>
using namespace std;
int main()
{
int i,time=0,num,found=0,loc;
int a[5];
for(i=0;i<5;i++)
{
cout<<"Put the value of numbers:"<<endl;
cin>>a[i];
}
cout<<"Enter the num which we want to search:"<<endl;
cin>>num;
for(i=0;i<5;i++)
{
time++;
if (a[i]==num)
{
found++;
loc=i;
break;
}
}
if(found==1)
cout<<"Number is found at:"<<loc<<endl;
else
cout<<"Not Found"<<endl;
cout<<"Your total time is:"<<time<<endl;
getche();
}
Binary Search:
The algorithm of binary search is much complex as compared with linear search. But it is a quick method to search a number in an array. If we find a number from an array of length 50, then we required our number at least after 6 comparisons.Binary search is applicable for only sorted array. You can't search a number from Binary Search in an unsorted array.
The basic algorithm of binary search is that, we firstly find a mid location of an array.
mid=beg+end/2;
After that we check that either a required number is greater than or smaller than mid location number. If the number is smaller then we move our end point at mid-1, and ignores the list which is greater than that of number.From that our list become half and then we repeat this method even that we not find the number.
#include<iostream>
#include<conio.h>
using namespace std;
int main()
{
int top,num,mid, bottom,flag,loc;
top=1;
bottom=9;
flag=0;
int arr[10]={1,2,3,4,5,6,7,8,9,10};
cout<<"Enter the number \t";
cin>>num;
while(top<=bottom && flag==0)
{
mid=(top+bottom)/2;
if(num<arr[mid])
{
bottom=mid-1;
loc=mid;
}
else if(num>arr[mid])
{
top=mid+1;
loc=mid;
}
else
{
flag=1;
loc=mid;
}
}
if(flag==1)
{
cout<<"Number found at loc \t"<<mid;
}
else
{
cout<<"numnber not found";
}
}